I've started trying to use Openai's chatbot as an replacement or at least an supplement to google search. I must admit so far it's provided me with far superior responses than a list of hits on websites for some specific keywords and phrases.
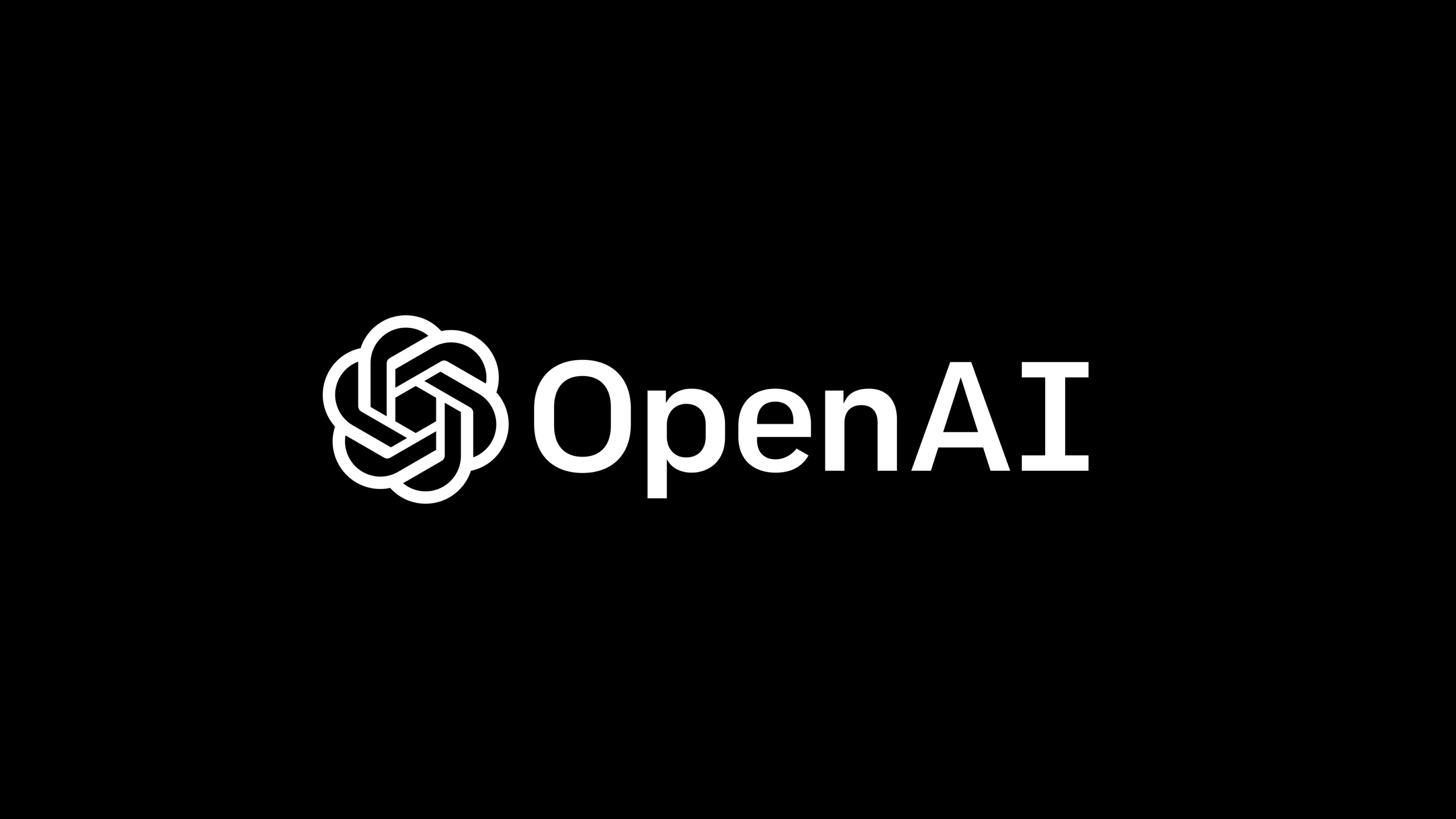
I think all developers without exception, uses google search on accession . It can greatly speed up your development time by utilizing google search for other peoples example project or q and a's or even just quickly get to the standard documentation for whatever particular subject you are working with, and its a great tool to have available, which I don't think anyone could live with out it to be honest, at least for now.
However I've started trying to use Openai's chatbot as an replacement or at least an supplement to google search. I must admit so far it's provided me with far superior responses than a list of hits on websites for some specific keywords and phrases.
In addition, my time spent researching a specific topic is also significantly smaller when utilizing openai chat compared to google search.
In openai chat, you have the option to actually ask elaborated questions and get a valid response formed in the way that you want, heck it can even translate code languages and give you working examples based on what you write.
Here's a simple of example of how you can utilize the features of openai chat. As you can see from the below dialog it can be quite the handy tool to utilize, I have also used the chat to convert typescript to c# code and similar other boiler plate tasks that we don't really need to spend time on as developers.
Seeing this makes me wonder how long it will be until developers no longer have to implement boiler plate code but simple implement the overarching architecture and structure and let the AI handled the implementation details based on what you describe.
Initial request
generate simple c# console game where the player can take multiple paths in the story
Response
using System;
namespace ConsoleGame
{
class Program
{
static void Main(string[] args)
{
// Introduction
Console.WriteLine("Welcome to the adventure game! You are a brave adventurer exploring a mysterious cave.");
Console.WriteLine("As you make your way deeper into the cave, you come to a fork in the path.");
Console.WriteLine("Do you want to go left or right? (Enter 'left' or 'right')");// Get player's choice
string choice = Console.ReadLine();// Check player's choice and branch the story based on it
if (choice.ToLower() == "left")
{
Console.WriteLine("You choose to go left. As you make your way down the left path, you come across a treasure chest.");
Console.WriteLine("Do you want to open the chest or leave it be? (Enter 'open' or 'leave')");// Get player's choice
string chestChoice = Console.ReadLine();if (chestChoice.ToLower() == "open")
{
Console.WriteLine("You decide to open the chest and find a treasure inside! You win!");
}
else
{
Console.WriteLine("You decide to leave the chest be and continue on your journey. The path leads you to a dead end.");
Console.WriteLine("You turn around and make your way back to the fork in the path. The end.");
}
}
else
{
Console.WriteLine("You choose to go right. As you make your way down the right path, you come across a deep chasm.");
Console.WriteLine("Do you want to try to cross the chasm or turn back? (Enter 'cross' or 'turn back')");// Get player's choice
string chasmChoice = Console.ReadLine();if (chasmChoice.ToLower() == "cross")
{
Console.WriteLine("You decide to try to cross the chasm. As you make your way across, you slip and fall to your death. Game over.");
}
else
{
Console.WriteLine("You decide to turn back. You make your way back to the fork in the path and try the other path. The end.");
}
}
}
}
}
Second request
refactor each step into a method
Response
using System;
namespace ConsoleGame
{
class Program
{
static void Main(string[] args)
{
Introduction();
string choice = GetChoice();
if (choice.ToLower() == "left")
{
LeftPath();
}
else
{
RightPath();
}
}static void Introduction()
{
Console.WriteLine("Welcome to the adventure game! You are a brave adventurer exploring a mysterious cave.");
Console.WriteLine("As you make your way deeper into the cave, you come to a fork in the path.");
Console.WriteLine("Do you want to go left or right? (Enter 'left' or 'right')");
}static string GetChoice()
{
return Console.ReadLine();
}static void LeftPath()
{
Console.WriteLine("You choose to go left. As you make your way down the left path, you come across a treasure chest.");
Console.WriteLine("Do you want to open the chest or leave it be? (Enter 'open' or 'leave')");string chestChoice = GetChoice();
if (chestChoice.ToLower() == "open")
{
Console.WriteLine("You decide to open the chest and find a treasure inside! You win!");
}
else
{
Console.WriteLine("You decide to leave the chest be and continue on your journey. The path leads you to a dead end.");
Console.WriteLine("You turn around and make your way back to the fork in the path. The end.");
}
}static void RightPath()
{
Console.WriteLine("You choose to go right. As you make your way down the right path, you come across a deep chasm.");
Console.WriteLine("Do you want to try to cross the chasm or turn back? (Enter 'cross' or 'turn back')");string chasmChoice = GetChoice();
if (chasmChoice.ToLower() == "cross")
{
Console.WriteLine("You decide to try to cross the chasm. As you make your way across, you slip and fall to your death. Game over.");
}
else
{
Console.WriteLine("You decide to turn back. You make your way back to the fork in the path and try the other path. The end.");
}
}
}
}